How to compare a synthetic CT and a CT?
You’d like to compare a CT and a synthetic CT you’ve produced from an MRI. Unless each DICOM object contains a way of distinguishing them in its description, the first step is to classify your data in different directories as follows:
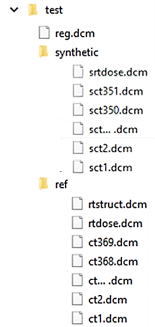
In the patient folder, create a sub-folder called “reference”, in which you will place the DICOM files of the CT, RTstruct and generated Rtdose. In the “synthetic” folder, you place the Rtdose generated from the synthetic CT and the synthetic CT files.
The REG matrix file is needed to link the synthetic CT and the CT reference.
The following script loads patient data into a list. It is then necessary to identify which items in the list belong to the “reference” folder and which belong to the “synthetic” folder. After loading the patient, we will create a list containing two elements (reference and synthetic), each containing DICOM objects (RTdose, RTstruct and scanner).
Feel free to run the script “step by step” (by placing your cursor on a line, then pressing CTRL + ENTER if you’re using RStudio).
library(espadon)
pat.dir <- "C:/data/my patient"
# patient loading
patient <- load.patient.from.dicom(pat.dir, data = TRUE)
# You can view the DICOM objects you have loaded:
display.obj.links(patient)
# List of reference and synthetic objects
pat <- lapply(c("reference", "synthetic"), function(dirname){
modality.idx <- (which(names(patient) == "T.MAT") + 1):length(patient)
obj.list <- lapply(modality.idx,function(idx){
patient[[idx]] [sapply(patient[[idx]], function(l) tolower(basename(l$file.dirname))) == dirname]
})
names(obj.list) <- names(patient)[modality.idx]
return( obj.list)
})
names(pat) <- c("reference", "synthetic")
# transfer matrix between the two geometric reference frames
tmat <- patient$T.MAT
# delete unnecessary data to free up memory space
rm(patient)
gc()
You can then inspect the RoI you can study by displaying part of the table given by pat[[“reference”]]$rtstruct[[1]]$roi.info
pat[["reference"]]$rtstruct[[1]]$roi.info[, c("name","roi.pseudo")]
name roi.pseudo
1 centering centering
2 EXTERNAL CONTOUR externalcontour
3 Bladder bladder
4 Rectum rectum
5 Sacrum sacrum
6 PTV ptv
We will choose here to calculate the metrics (Mean Error, Mean Absolute Error, Mean Squared Error, Root Mean Squared Error) of the RoI “Sacrum”, “Rectum”, “Bladder ” and “PTV ”.
roi.to.analyze.name <- c("Sacrum","Rectum", Bladder ", "PTV "),
roi.to.analyze.idx <- select.names(pat[["reference"]]$rtstruct[[1]]$roi.info$roi.pseudo,
roi.name = roi.to.analyze.name)
metrics <- err.metrics.from.roi(obj = pat$synthetic$ct[[1]],
obj.ref = pat$reference$ct[[1]],
struct = pat$synthetic$rtstruct[[1]],
roi.name = roi.to.analyze,
roi.sname = NULL, roi.idx = NULL,
T.MAT = tmat)
Note that you could also have calculated the metrics using roi.sname or roi.idx, or by mixing roi.name, roi.sname and roi.idx.
The 3 uses of the err.metrics.from.roi function below are equivalent:
metrics <- err.metrics.from.roi(obj = pat$synthetic$ct[[1]],
obj.ref = pat$reference$ct[[1]],
struct = pat$reference$rtstruct[[1]],
roi.name = NULL,
roi.sname = c("sacr","rectum",
"bladd", "ptv"),
roi.idx = NULL, .MAT = tmat)
# or
metrics <- err.metrics.from.roi(obj = pat$synthetic$ct[[1]],
obj.ref = pat$reference$ct[[1]],
struct = pat$reference$rtstruct[[1]],
roi.name = NULL, roi.sname = NULL,
roi.idx = c (3,4,5,6), T.MAT = tmat)
# or
metrics <- err.metrics.from.roi(obj = pat$synthetic$ct[[1]],
obj.ref = pat$reference$ct[[1]],
struct = pat$reference$rtstruct[[1]],
roi.name = NULL, roi.sname = NULL,
roi.idx = roi.to.analyze.idx, T.MAT = tmat)
You can inspect the metrics variable
metrics
ROI ME MAE MSE RMSE
1 Bladder 12.427748 17.52306 539.189 23.22044
2 Rectum 7.496449 33.16230 6120.671 78.23472
3 Sacrum -67.590704 152.87903 51226.703 226.33317
4 PTV 19.384144 45.51664 16568.974 128.72053
then save it in a *.csv file in the patient directory with the instruction :
write.csv2 (metrics, file.path (pat.dir, paste(basename(pats.dir), "metrics.csv")),
row.names = FALSE)